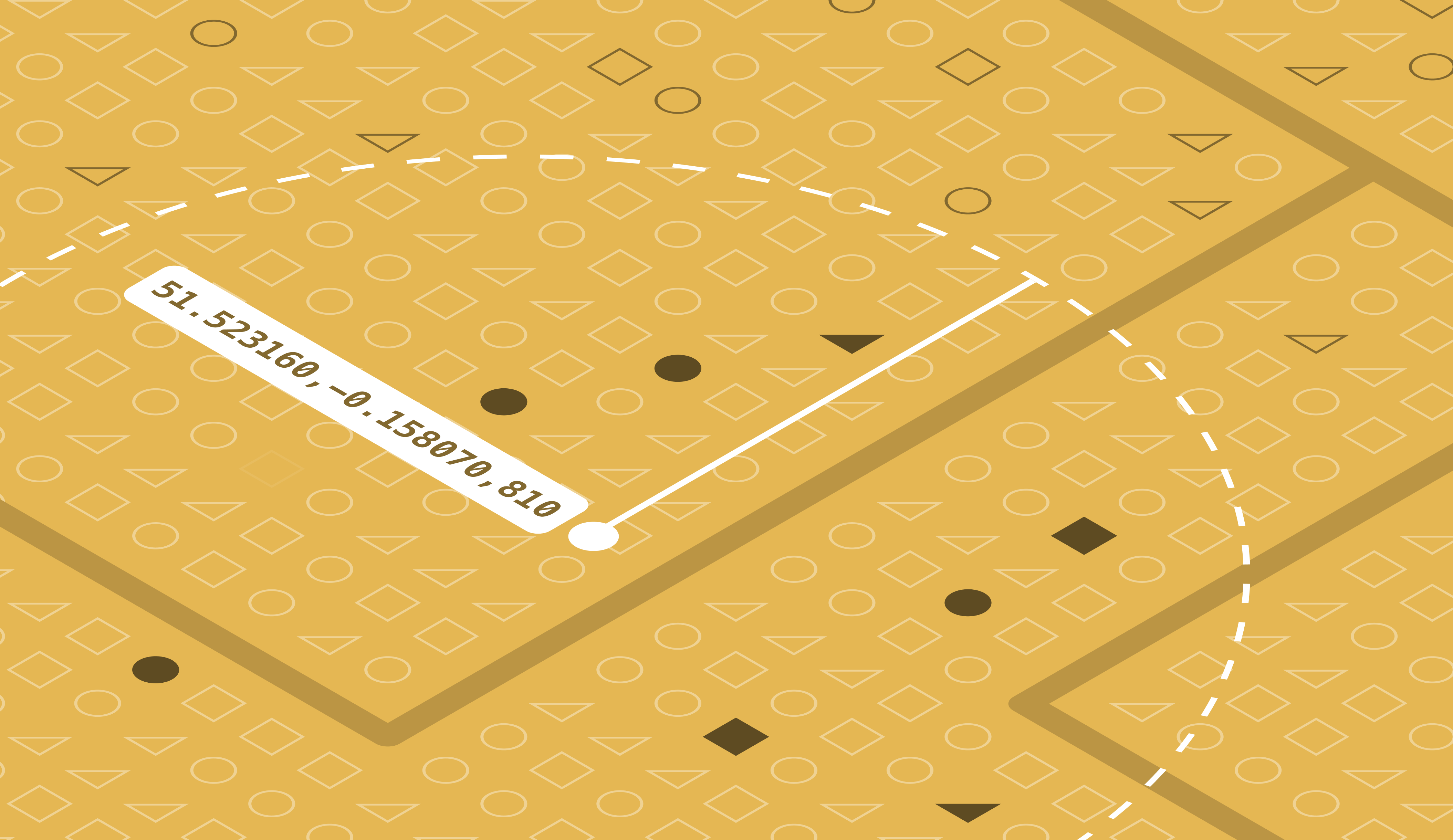
Filter Your Search Results by Geography — Now in Private Beta!
Hot on the heels of Text Highlights last week, the team here at Objective HQ is shipping Geo-filtering into self-service Private Beta today! Geo-filtering gives your app the ability to filter search results by a geographic location (only Earth coordinates are supported currently 🌎). This allows you to surface both semantically relevant and location-aware search results to your users when geography is important, such as brick-and-mortar store searches, concert event searches, and job searches. If you already have a Private Beta account, go check it out in your Console now! If you aren’t, jump on the waitlist — we’re still onboarding developers as fast as we can, and we’ll let you know when your account is ready!
Your search app just learned how to speak latitude & longitude!
Like everything in Objective, Geo-filtering is a simple-but-powerful way to filter search results by radial proximity to a location of interest. You can implement Geo-filtering by adding geographic data to their objects and configuring your search indices to recognize this data for geo-based queries. You can then filter results by distance in meters from a lat/long point using:
filter_query={yourFieldName}:@{latitude},{longitude},{distanceInMeters}
Let’s write some code!
We’ll be using objective-sdk
in Python today, but you can build along with us in Typescript if that’s your flavor (grab our Typescript library here!).
As always, first thing’s first — if you haven’t installed objective-sdk
yet, grab it on PyPi:
Now — like we talked about earlier, you can geo-enable all of the Objects in your Object Store simply by adding a field with latitude and longitude coordinates as floats to your Objects. Let’s say we’re building restaurant search. Our objects might look like this (what do you mean you’ve never been to Joan’s Giant Burgers in London?):
Pretty simple, right? Then, adding those Objects to our Object Store looks something like this:
Next, we’ll need to create an Index that is configured with our store_coordinates
added to the filterable
array, so that Objective Search knows to use it in filtering. And we have to let Objective know that store_coordinates
is a “geo” field type. That looks like this:
And now we just have to do some searching — which we already know is the easiest part of using Objective. All we have to add to our query to start Geo-filtering is the filter_query parameter, with the coordinates and a distance as measured in meters:
Let’s take a closer look at that filter string, as there are a few things going on here:
The geo-filter is a string that can be broken down into these component parts:
{yourFieldName}:@{latitude},{longitude},{distanceInMeters}
So in our example, we’re searching within 810 meters (about half a mile - we don’t want to walk forever, we’re hungry) of 221B Baker St. in London — the infamous address of one of our favorite searchers & finders, Sherlock Holmes. It’s a tourist trap, we know. But you can’t not visit when you’re in the neighborhood.
And as it turns out, Joan’s Giant Burgers is the only spot within a quick walk nearby. You’ll receive all relevant search results from the .search()
method call, with any Objects filtered out whose distance is further than the 810 meters we specified.
You can start to imagine how powerful a tool this can be when you’re building search experiences that need to be location aware. From searching for retail stores, to searching for events that are connected to a physical space — like a concert. Or helping users find jobs that connected to a company’s physical office, within a comfortable driving distance for the user.
Like with all of Objective, the only real limit is your imagination. And we can’t wait to see what you build!